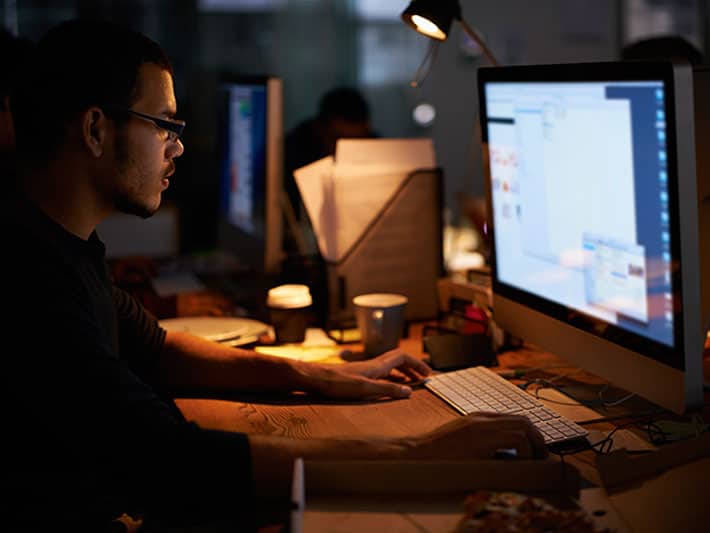
A junior web developer can code fast, but will often make many more mistakes. Some errors in logic can be, but especially methodological errors, which can waste a lot of time and make the maintenance of the application much more difficult. The mistakes of a junior web developer are often the same: lack of planning, refusal to give up, etc.
We relied on an article published by Samer Buna on Medium to offer you a tour of the 20 classic mistakes of a junior web developer. A must-read for any junior web developer, but also for the people who work with them.
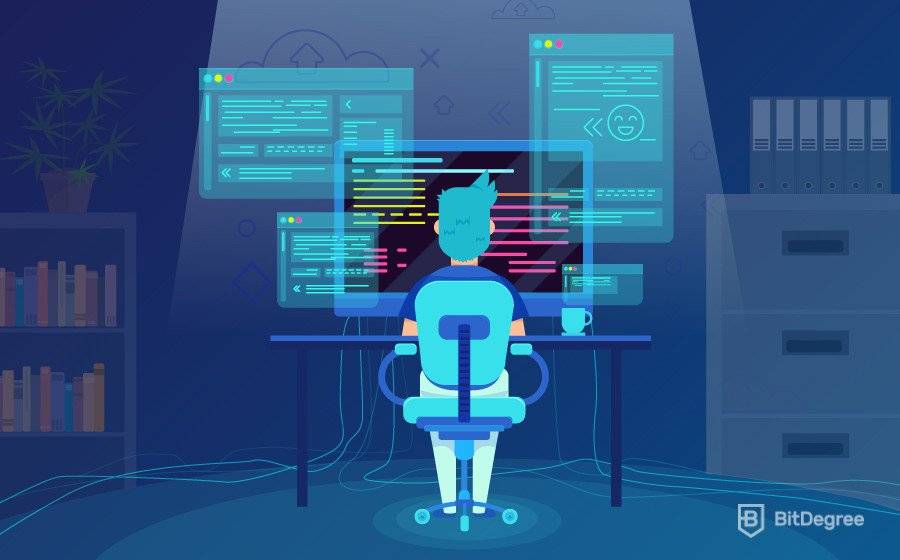
1. Write the code without planning anything
In general, high-quality editorial content cannot be created easily. It requires careful thought and research. The creation of computer programs is no exception. Writing quality programs is a process where you have to take steps: think, research, plan, write, validate, modify. Unfortunately, there is no good acronym for this. One of the most common mistakes as a newbie programmer is starting to write code without much thought and research. While this might work for a small standalone app, it does harm larger apps. Just as you have to think before you say something that you might regret, you should think before you code. Coding is also a way to communicate your thoughts. Programming is mostly about reading the previous code, figuring out what is needed and how to fit it into the current system, and planning to write functions with small additions. The actual writing of lines of code is only 10% of the whole process. Programming is a creativity based on logic not just lines of code.
2. Plan too much before writing the code
Yes, planning before you dive into writing code is good, but even good things can do you a disservice when you overdo it. Don’t look for a perfect plan, it doesn’t exist in the programming world. Your plan will change along the way anyway, but what it’s good for is forcing you to stay structured. This leads to more clarity in your code. Too much planning is a waste of time. We’re only talking about planning for small features. This is what we call the waterfall approach, which is a linear plan with separate stages that must be completed one by one.
Writing programs should be a reactive activity. You’ll add features you never would have thought of in a waterfall plan. You have to fix the bugs and adapt to the changes. However, you should plan your next features to a minimum. Do this very carefully because too little planning and too much planning can adversely affect the quality of your code. You have to choose the right dosage.
3. Underestimate the importance of code quality
If you can only focus on one aspect of the code, it should be its readability. An unclear code will go straight to the trash. Never underestimate the importance of code quality. Your main job as a coder is to communicate the implementations of all the solutions you are working on. Even the little things matter. For example, if you are not consistent with your indentation, you should just stop the code.
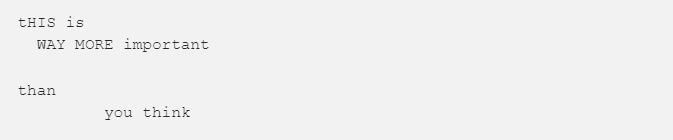
Another simple thing is the use of long lines. Anything over 80 characters is much more difficult to read. You might be tempted to put a long condition on the same line to keep a block more visible, that’s a mistake. Never go beyond the 80 character limit. Lots of simple problems like this can be easily solved with formatting tools. In JavaScript, we have two great tools that work perfectly together: ESLint and Prettier. Here are some other errors related to the quality of the code:
- Use multiple lines in a function or a file. You should always break long code into smaller pieces that can be tested and managed separately.
- Use of short, generic variable names. Give your variables descriptive and unambiguous names.
- Use shortcuts and workarounds to avoid spending more time around simple problems.
- Thinking that the more code the better. A shorter code is better in most cases. Write longer versions only if they make the code more readable. For example, don’t use smart quotes and expressions just to keep the code shorter. Removing unnecessary code is the best thing you can do in any program.
4. Choose the first solution
While the first solution you find may be tempting, the best solutions are usually discovered as soon as you start to look at all the possible solutions. If you can’t find more than one solution to a problem, it’s probably a sign that you don’t fully understand the problem. Your job as a programmer is not to find a solution to the problem, but to find THE easiest solution to the problem. By “simple” we mean that the solution must work well and perform well, while still being simple enough to read, understand and maintain.
5. The “non-abandonment”
Another common mistake is to stick with the first solution even after identifying that it may not be the easiest approach. This is probably psychologically related to the “no-give up” mentality. It’s a good mindset to have in most activities, but it shouldn’t apply to programming. When it comes to programming, the “right” mindset often fails. As soon as you start to doubt a solution, you should consider rethinking the problem, no matter how much you have invested in that solution. Source control tools like GIT can help you experiment with many different solutions.
6. Don’t use Google
There are surely times when you have wasted time when you could have searched for the solution on Google. Unless you are using cutting-edge technology, when you have a problem there is a good chance that someone else will encounter the same problem and find a solution. Save yourself some time and use Google. Sometimes Google reveals that what you thought was a problem isn’t real, and what you need to do is not fix it, but rather adapt it. Don’t assume that you know everything about choosing a solution to a problem. Google will surprise you. However, be careful what you search for on Google. Another sign of a beginner is copying and using code as is without understanding it. Although this code may correctly resolve your problem,
7. Plan for the future
It is often tempting to think beyond the solution you write. All kinds of scenarios will pop up in your head with every line of code you write. But it would be a mistake to use this in each case. Don’t write code that you don’t need today. Writing a feature because you think you might need it in the future is just pointless. Always write the minimum amount of code you need today for the solution you are implementing.
8. Not using the right data structures
When preparing for interviews, novice programmers usually put too much emphasis on algorithms. It’s good to identify good algorithms and use them when needed, but memorizing them won’t help your genius. However, memorizing the strengths and weaknesses of the different data structures that you can use in your language will certainly make you a better developer. This article is not intended to teach you about data structures, but here are some examples:
Using lists (tables) instead of cards (objects) to manage records
Probably the most common data structure error is using lists instead of cards to manage a list of records. We are talking here about a list of records where each record has an identifier that should be used to search for that record. In JavaScript, the most common list structure is an array and the most common map structure is an object (there is also a map structure in modern JavaScript). The use of lists on cards to manage records is often wrong. The main reason this is important is that when you search for records using their IDs, cards are much faster than lists.
Do not use stacks
When writing code that requires some form of recursion, it is always tempting to use simple recursive functions. However, it is generally difficult to optimize recursive code, especially in single-threaded environments. For example, optimizing a recursive function that returns two or more calls to itself is much more difficult than optimizing a recursive function that simply returns a single call to itself. What we tend to overlook as newbies is that there is an alternative to using recursive functions. You can just use a stack structure.
9. Write comments on obvious things
Most comments can be replaced with better-named elements in your code. For example, instead of the following code:
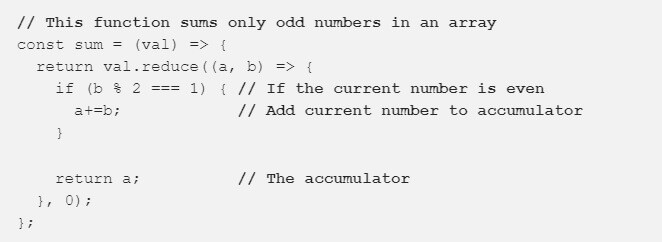
The same code can be written without comments like this:
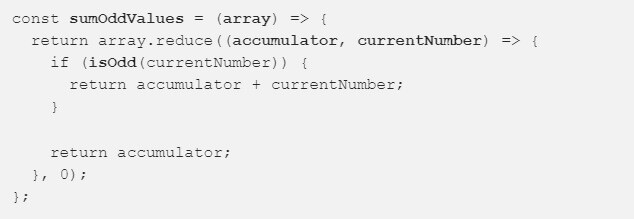
Just using better names for functions and arguments makes most comments unnecessary. Sometimes you’re still forced into situations where the only clarity you can add to the code is comments. This is when you need to structure your comments to answer the question of WHY this code rather than the question of WHAT does this code do? Here is an example of unnecessary comments that only add glitches to the code:
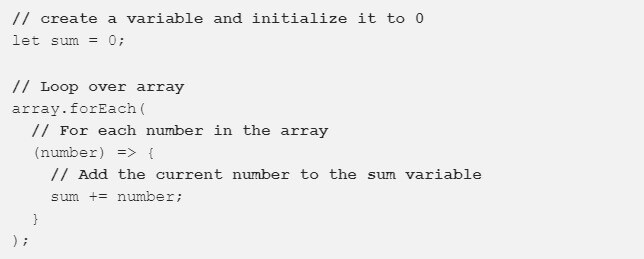
Don’t become that programmer. Do not accept this code. Remove these comments if you need to process them. If you employ programmers who write reviews like these, ask yourself the right questions.
10. Don’t write tests
If you think you are an expert programmer and your thinking gives you the confidence to write code without testing, you are a beginner. If you don’t write tests in code, you’ll probably be testing your program in another way, manually. If you’re building a web app, you’ll refresh and interact with the app after each line of code. There is nothing wrong with manually testing your code. However, you must manually test your code to determine how to test it automatically. If you successfully test an interaction with your application, you should go back to your editor and write code to automatically perform the same interaction the next time you add code to the project. You are a human being. You will forget to test all previously successful validations after each code change. Let a computer do it. If you can, guess or design your validations first before you even write the code to satisfy them. Test-Driven Development (TDD) isn’t just a “hype”. It positively affects how you think about your features and how to create a better design for them. TDD isn’t for everyone and it doesn’t work well for all projects, but if you can use it (even partially) you should. Test-Driven Development (TDD) isn’t just a “hype”. It positively affects how you think about your features and how to create a better design for them. TDD isn’t for everyone and it doesn’t work well for all projects, but if you can use it (even partially) you should. Test-Driven Development (TDD) isn’t just a “hype”. It positively affects how you think about your features and how to create a better design for them. TDD isn’t for everyone and it doesn’t work well for all projects, but if you can use it (even partially) you should.
11. Do not question the existing code
Unless you are a great coder who always works solo, there is no doubt that you will come across headless codes in your life. Newbies won’t recognize it and they usually assume it’s good code because it seems to work and it’s been in the code for a long time. What’s worse is that if the bad code uses bad practices, the beginner might be tempted to repeat the bad practice elsewhere in the code. Some code looks bad, but it may have a special condition that requires the developer to write it this way. As a beginner, you should just assume that any undocumented code that you don’t understand is more likely to be bad. If the author of this code is long gone or no longer remembers it, look for this code and try to figure it all out. It is only when you fully understand the code that you will come to a judgment of whether it is good or not.
12. Observe best practices
The term “best practice” is damaging. This implies that no further research is necessary. There are no best practices. There are probably good practices at time T and for this programming language. Some of what we previously identified as best programming practices are now called bad practices. You can always find better practices if you invest enough time. Stop worrying about best practices and focus on what you can do best. Don’t do something because of a quote you’ve read somewhere, or because you’ve seen someone else do it, or because someone said it’s good practice. Challenge all theories,
13. Obsession with performance
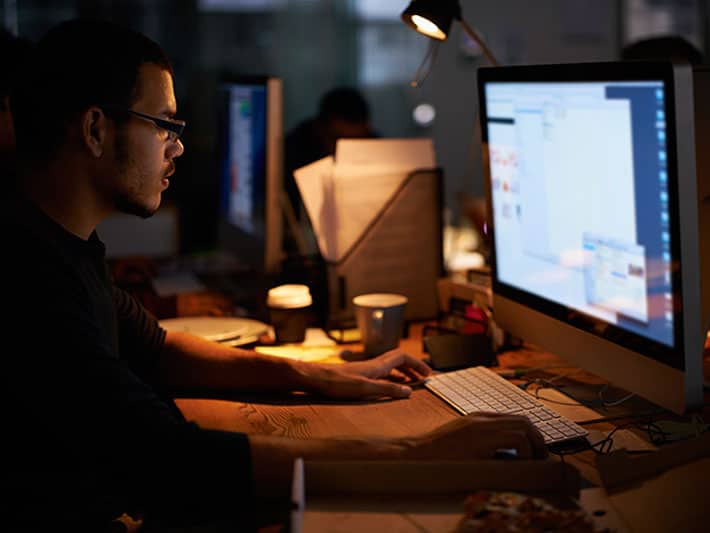
While the lineup has changed significantly since Donald Knuth wrote the above statement, it still contains valuable advice today. The good rule of thumb to remember about this is: If you can’t measure the performance problem with the code, don’t try to optimize it. If you optimize before running the code, there’s a good chance you’ll be doing it prematurely. There is also a great chance that the optimization you are doing is completely unnecessary. Any unobvious optimization that is performed on existing code without measurements is considered harmful and should be avoided. What you think is a performance boost, could turn out to be a source of unexpected new bugs. Don’t waste your time optimizing unmeasured performance issues.
14. Do not target the end-user experience
What’s the easiest way to add functionality to an app? Look at it from your perspective and how it fits into the current user interface. Be one of those developers who put themselves in their users’ shoes. They imagine what the users of this feature need and how they can behave. They think about ways to make the feature easy to find and use, not how to make the feature exist without any thought about whether or not to use that feature.
“Premature optimization is the root of all evil in programming”
Donald Knuth (1974)
15. “Reinventing the wheel”
This is a delicate point. Programming is not a well-defined field. Things change very quickly and new requirements are introduced very quickly. For example, if you need a wheel that spins at different speeds depending on the time of day, instead of customizing the wheel we all know maybe we need to rethink it. The great thing about software “wheels” is that most of them are free and open so you can see their internal design. You can easily judge the coding wheels based on their internal design quality. Use open-source wheels if you can which can be debugged and fixed easily. Don’t include an entire library just to use one or two functions. The best example of this is the dash library in JavaScript. If you just need to shuffle an array, you just need to import the shuffle method. Do not import the entire lodash library.
16. Have the wrong attitude towards reviews on your code
One sign of a newbie coder is that they often view code reviews as criticism. If you feel this way, you need to change that attitude right away. Look at every code review as a learning opportunity. And, the most important thing is to have constructive criticism. In the code, you will learn about it every day. Most reviews will tell you something you didn’t know. Sometimes the review won’t be timely and it will be your turn to teach them something. However, if something is not evident from your code then maybe it needs to be changed. If you need to learn something from those who criticize you, just know that teaching is one of the most rewarding things you can do as a programmer.
17. Don’t use source code control
Beginners sometimes underestimate the power of a good source/revision control system. Source control isn’t just about pushing your changes for others to develop. Source control is about clear history. The code will be challenged, and the history of the progress of this code will help answer some of the more difficult questions. This is another channel for communicating your implementations and helps future maintainers of your code understand how the code reached the state it is currently in. Be specific with your posts, but keep in mind that they should be summaries. Do not include anything unnecessary in your validation messages. For example, do not list files that have been added, changed, or deleted in your summaries. Source control is also about discoverability. If you come across a function and start questioning its need or design, you can find the commit that introduced it and see the context of that function. Validations can even help you identify which code introduced a bug to the program. Git even offers a binary search in commits (the bisect command) to locate the only culprit commit that introduced a bug.
18. Overuse of shared state programming
The shared state is a source of problems and should be avoided, if possible. If this is not possible, the use of a shared state should be kept to a minimum. As a newbie programmer, you might not have realized that every variable we set represents a shared state. It contains data that can be modified by all elements in the same scope as this variable. The more global the scope, the worse the duration of this shared state. The big problem with a shared state starts when multiple resources have to change that state together in the same event loop.
19. Have the wrong attitude about mistakes
Mistakes are a good thing, they mean you are making progress. Expert programmers love mistakes, newbies hate them. If you are bothered by seeing these wonderful little red error messages, you need to change your attitude. You have to look at them as helpers and you have to use them to progress. Some errors should be regarded as exceptions. Exceptions are user-defined errors that you must schedule. Some errors should be left. They need to crash the app to get them out.
20. Don’t take breaks
You are human and your brain needs breaks. It is not something that you can compromise. Build something into your workflow to force yourself to take breaks. Take lots of breaks, but shorter. Leave your desk and take a short walk. Use this moment to think about what to do next. Get back to code with brand new eyes.
We hope this article has helped you avoid falling into the common pitfalls when you are a junior developer. Do you have any other tips to share with us? Do not hesitate to leave us a little comment below.